Logging custom events
Learn how to log custom events through the Braze SDK.
note:
For wrapper SDKs not listed, use the relevant native Android or Swift method instead.
Logging a custom event
To log a custom event, use the following event-logging method.
For native Android, you can use the following method:
1
| Braze.getInstance(context).logCustomEvent(YOUR_EVENT_NAME);
|
1
| Braze.getInstance(context).logCustomEvent(YOUR_EVENT_NAME)
|
1
| AppDelegate.braze?.logCustomEvent(name: "YOUR_EVENT_NAME")
|
1
| [AppDelegate.braze logCustomEvent:@"YOUR_EVENT_NAME"];
|
For a standard Web SDK implementation, you can use the following method:
1
| braze.logCustomEvent("YOUR_EVENT_NAME");
|
If you’d like to use Google Tag Manager instead, you can use the Custom Event tag type to call the logCustomEvent
method and send custom events to Braze, optionally including custom event properties. To do this:
- Enter the Event Name by either using a variable or typing an event name.
- Use the Add Row button to add event properties.
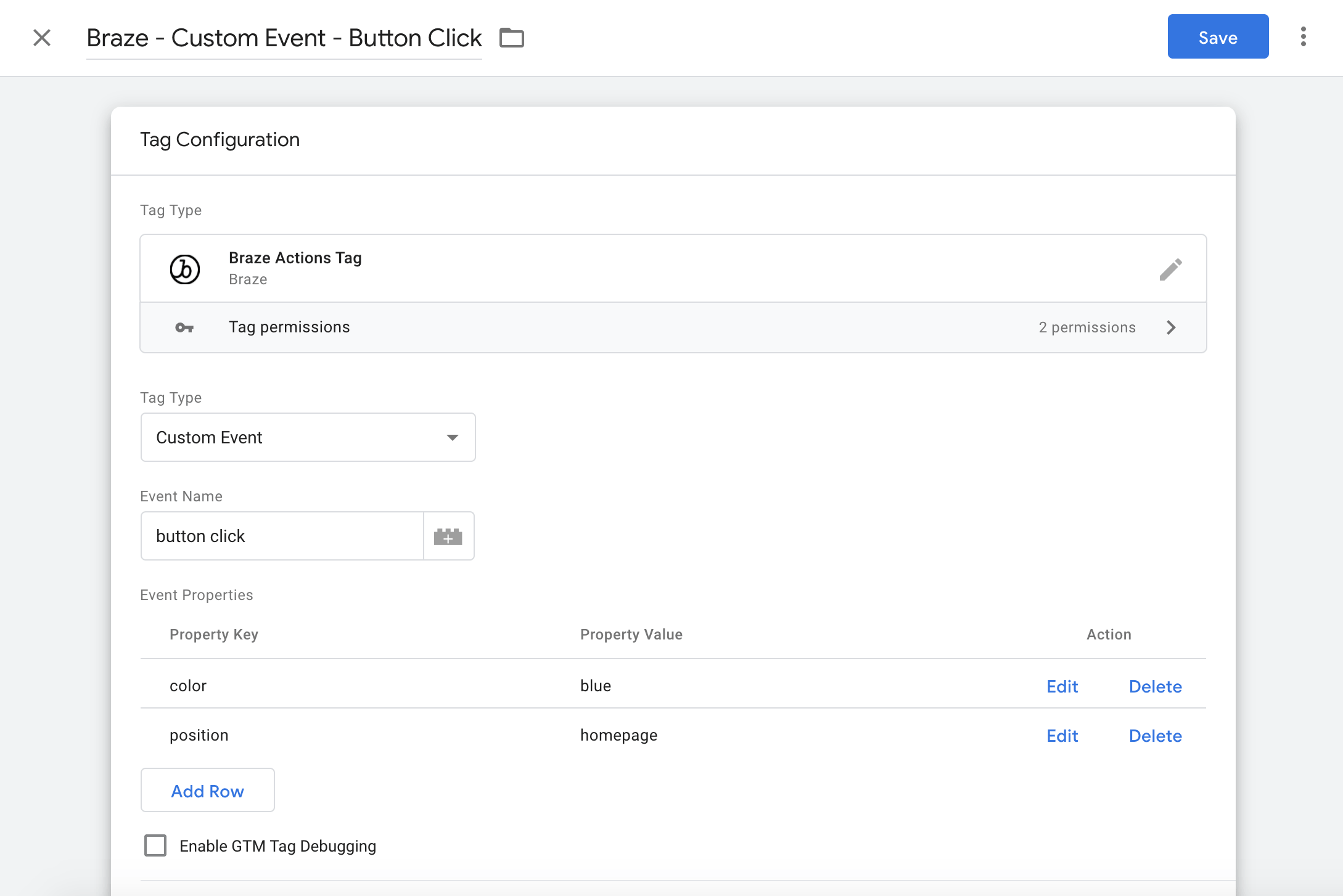
1
| braze.logCustomEvent('YOUR_EVENT_NAME');
|
If you’ve integrated Infillion Beacons into your Android app, you can optionally use visit.getPlace()
to log location-specific events. requestImmediateDataFlush
verifies that your event will log even if your app is in the background.
1
2
| Braze.getInstance(context).logCustomEvent("Entered " + visit.getPlace());
Braze.getInstance(context).requestImmediateDataFlush();
|
1
2
| Braze.getInstance(context).logCustomEvent("Entered " + visit.getPlace())
Braze.getInstance(context).requestImmediateDataFlush()
|
1
| Braze.logCustomEvent("YOUR_EVENT_NAME");
|
1
| m.Braze.logEvent("YOUR_EVENT_NAME")
|
1
| AppboyBinding.LogCustomEvent("YOUR_EVENT_NAME");
|
1
| UBraze->LogCustomEvent(TEXT("YOUR_EVENT_NAME"));
|
When you log a custom event, you have the option to add metadata about that custom events by passing a properties object with the event. Properties are defined as key-value pairs. Keys are strings and values can be string
, numeric
, boolean
, or Date
objects.
To add metadata properties, use the following event-logging method.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
| Braze.logCustomEvent("YOUR-EVENT-NAME",
new BrazeProperties(new JSONObject()
.put("you", "can")
.put("pass", false)
.put("orNumbers", 42)
.put("orDates", new Date())
.put("or", new JSONArray()
.put("any")
.put("array")
.put("here"))
.put("andEven", new JSONObject()
.put("deeply", new JSONArray()
.put("nested")
.put("json"))
)
));
|
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
| Braze.logCustomEvent("YOUR-EVENT-NAME",
BrazeProperties(JSONObject()
.put("you", "can")
.put("pass", false)
.put("orNumbers", 42)
.put("orDates", Date())
.put("or", JSONArray()
.put("any")
.put("array")
.put("here"))
.put("andEven", JSONObject()
.put("deeply", JSONArray()
.put("nested")
.put("json"))
)
))
|
1
2
3
4
5
6
7
8
9
10
11
12
13
| AppDelegate.braze?.logCustomEvent(
name: "YOUR-EVENT-NAME",
properties: [
"you": "can",
"pass": false,
"orNumbers": 42,
"orDates": Date(),
"or": ["any", "array", "here"],
"andEven": [
"deeply": ["nested", "json"]
]
]
)
|
1
2
3
4
5
6
7
8
9
10
11
| [AppDelegate.braze logCustomEvent:@"YOUR-EVENT-NAME"
properties:@{
@"you": @"can",
@"pass": @(NO),
@"orNumbers": @42,
@"orDates": [NSDate date],
@"or": @[@"any", @"array", @"here"],
@"andEven": @{
@"deeply": @[@"nested", @"json"]
}
}];
|
1
2
3
4
5
6
7
8
9
10
| braze.logCustomEvent("YOUR-EVENT-NAME", {
you: "can",
pass: false,
orNumbers: 42,
orDates: new Date(),
or: ["any", "array", "here"],
andEven: {
deeply: ["nested", "json"]
}
});
|
1
2
3
4
5
| braze.logCustomEvent('custom_event_with_properties', properties: {
'key1': 'value1',
'key2': ['value2', 'value3'],
'key3': false,
});
|
1
2
3
4
5
| Braze.logCustomEvent("custom_event_with_properties", {
key1: "value1",
key2: ["value2", "value3"],
key3: false,
});
|
1
| m.Braze.logEvent("YOUR_EVENT_NAME", {"stringPropKey" : "stringPropValue", "intPropKey" : Integer intPropValue})
|
1
| AppboyBinding.LogCustomEvent("event name", properties(Dictionary<string, object>));
|
1
2
3
4
5
6
7
8
9
| TMap<FString, FString> Properties;
Properties.Add(TEXT("you"), TEXT("can"));
Properties.Add(TEXT("pass"), TEXT("false"));
Properties.Add(TEXT("orNumbers"), FString::FromInt(42));
Properties.Add(TEXT("orDates"), FDateTime::Now().ToString());
Properties.Add(TEXT("or"), TEXT("any,array,here")); // Arrays are stored as comma-separated strings
Properties.Add(TEXT("andEven"), TEXT("deeply:nested,json"));
UBraze->LogCustomEventWithProperties(TEXT("YOUR_EVENT_NAME"), Properties);
|
important:
The time
and event_name
keys are reserved and cannot be used as custom event properties.